When working with a Next.js project, you might encounter the following error while attempting to launch your development server:
Error: Configuring Next.js via 'next.config.ts' is not supported. Please replace the file with 'next.config.js' or 'next.config.mjs'.
at loadConfig (/path/to/your/project/node_modules/next/dist/server/config.js)
at async Module.nextDev (/path/to/your/project/node_modules/next/dist/cli/next-dev.js)
at loadConfig (/path/to/your/project/node_modules/next/dist/server/config.js)
at async Module.nextDev (/path/to/your/project/node_modules/next/dist/cli/next-dev.js)
Let's fix this error by changing the Next.js configuration file from TypeScript to JavaScript format.
- First, if you have a
next.config.ts
file, remove it:
rm next.config.ts
- Create a new
next.config.js
file:
cat > next.config.js << 'EOL'
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
swcMinify: true,
}
module.exports = nextConfig
EOL
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
swcMinify: true,
}
module.exports = nextConfig
EOL
- Also, let's fix the tailwind config since we're using JavaScript:
# Remove the TypeScript version if it exists
rm tailwind.config.ts
rm tailwind.config.ts
# Create JavaScript version
cat > tailwind.config.js << 'EOL'
/** @type {import('tailwindcss').Config} */
module.exports = {
darkMode: ["class"],
content: [ './pages/**/*.{ts,tsx}',
'./components/**/*.{ts,tsx}', './app/**/*.{ts,tsx}', './src/**/*.{ts,tsx}', ], theme: { container: { center: true, padding: "2rem", screens: { "2xl": "1400px", }, }, extend: { keyframes: { "accordion-down": { from: { height: 0 }, to: { height: "var(--radix-accordion-content-height)" }, }, "accordion-up": { from: { height: "var(--radix-accordion-content-height)" }, to: { height: 0 }, }, }, animation: { "accordion-down": "accordion-down 0.2s ease-out", "accordion-up": "accordion-up 0.2s ease-out", }, }, }, plugins: [require("tailwindcss-animate")], } EOL
cat > tailwind.config.js << 'EOL'
/** @type {import('tailwindcss').Config} */
module.exports = {
darkMode: ["class"],
content: [ './pages/**/*.{ts,tsx}',
'./components/**/*.{ts,tsx}', './app/**/*.{ts,tsx}', './src/**/*.{ts,tsx}', ], theme: { container: { center: true, padding: "2rem", screens: { "2xl": "1400px", }, }, extend: { keyframes: { "accordion-down": { from: { height: 0 }, to: { height: "var(--radix-accordion-content-height)" }, }, "accordion-up": { from: { height: "var(--radix-accordion-content-height)" }, to: { height: 0 }, }, }, animation: { "accordion-down": "accordion-down 0.2s ease-out", "accordion-up": "accordion-up 0.2s ease-out", }, }, }, plugins: [require("tailwindcss-animate")], } EOL
- Make sure your
components.json
matches these changes:
cat > components.json << 'EOL' { "$schema": "https://ui.shadcn.com/schema.json", "style": "default", "rsc": true, "tsx": true, "tailwind": { "config": "tailwind.config.js", "css": "app/globals.css", "baseColor": "slate", "cssVariables": true }, "aliases": { "components": "@/components", "utils": "@/lib/utils" } } EOL
Now try running the development server again:
npm run dev
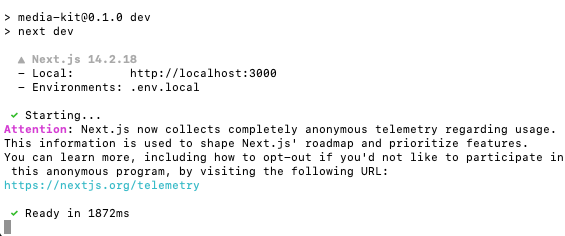
You are now all set ! Time to code 😄