In the fast-paced world of streaming, a well-designed media kit is essential for showcasing your brand and engaging potential sponsors. A media kit acts as a resume for streamers, highlighting key metrics, audience demographics, and brand partnerships in one cohesive and visually appealing package. But what if your media kit could automatically pull live Twitch data, such as follower counts, average viewers, and recent streams?
In this article, we’ll guide you through the process of building a dynamic media kit page using React. By integrating Twitch’s API, we’ll create a media kit that updates in real-time, providing streamers with accurate and up-to-date insights without manual input. Whether you’re looking to build your own media kit platform or just want to enhance your online presence, this approach combines technical flexibility with powerful data visualization.
First, let's set up the project locally:
- Open Terminal and create a new Next.js project:
When prompted, use these settings:
- Would you like to use TypeScript? Yes
- Would you like to use ESLint? Yes
- Would you like to use Tailwind CSS? Yes
- Would you like to use
src/
directory? Yes - Would you like to use App Router? Yes
- Would you like to use Turbopack? › No
- Would you like to customize the import alias (@/* by default)? › No
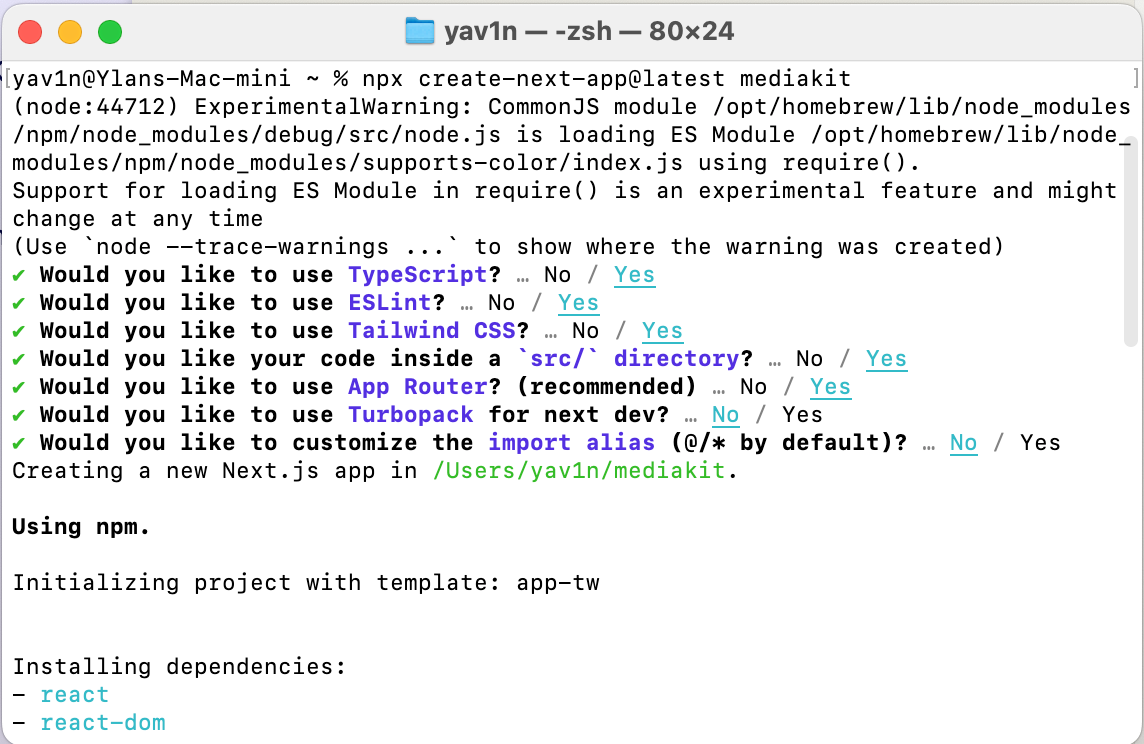
- Install additional dependencies:
If your version React is 19 or higher, you might encounter an error at this stage of the setup.
npm error code ERESOLVE
npm error ERESOLVE unable to resolve dependency tree
npm error
npm error While resolving: mediakit@0.1.0
npm error Found: react@19.0.0-rc-66855b96-20241106
npm error node_modules/react
npm error react@"19.0.0-rc-66855b96-20241106" from the root project
npm error
npm error Could not resolve dependency:
npm error peer react@"^16.0.0 || ^17.0.0 || ^18.0.0" from recharts@2.13.3
npm error node_modules/recharts
npm error recharts@"*" from the root project
npm error
npm error Fix the upstream dependency conflict, or retry
npm error this command with --force or --legacy-peer-deps
npm error to accept an incorrect (and potentially broken) dependency resolution.
npm error
npm error
npm error For a full report see:
npm error /Users/yav1n/.npm/_logs/2024-11-29T01_06_45_871Z-eresolve-report.txt
npm error A complete log of this run can be found in: /Users/yav1n/.npm/_logs/2024-11-29T01_06_45_871Z-debug-0.log
This error occurs because the project is using React 19 (canary/experimental version) while some of our dependencies require React 16-18. Let's modify the installation command to handle these dependency conflicts.
Use this command instead:
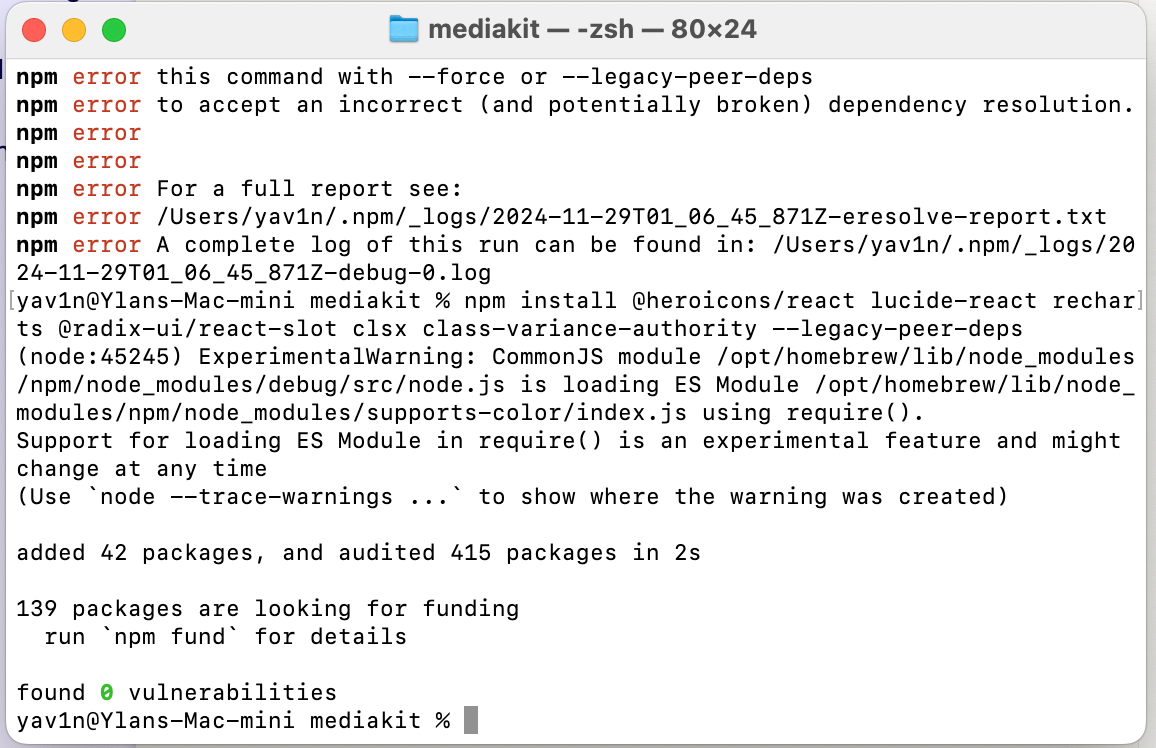
- Create the main component structure. Create a new directory and file:
touch src/components/MediaKitPage.tsx

- Update the main page file to use our new component
- We copied component code into
src/components/file.tsx
Since Recharts does not work with React 19, we needed to make some changes
Let's do a complete reset to fix this issue:
- First, delete these files/folders:
rm package-lock.json
- Update your
package.json
. Replace the entire content with:
{
"name": "mediakit",
"version": "0.1.0",
"private": true,
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"lint": "next lint"
},
"dependencies": {
"@heroicons/react": "^2.0.18",
"@radix-ui/react-slot": "^1.0.2",
"class-variance-authority": "^0.7.0",
"clsx": "^2.0.0",
"lucide-react": "^0.292.0",
"next": "14.0.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"recharts": "^2.9.3"
},
"devDependencies": {
"@types/node": "^20.10.0",
"@types/react": "^18.2.39",
"@types/react-dom": "^18.2.17",
"autoprefixer": "^10.4.16",
"eslint": "^8.54.0",
"eslint-config-next": "14.0.3",
"postcss": "^8.4.31",
"tailwindcss": "^3.3.5",
"typescript": "^5.3.2"
}
}
- Reinstall all dependencies:
- Make sure your
src/app/page.tsx
contains:
import MediaKitPage from '@/components/MediaKitPage';
export default function Home() {
return <MediaKitPage />;
}
Server Error
Error: Super expression must either be null or a function
This error happened while generating the page. Any console logs will be displayed in the terminal window.
Call Stack
_inherits
node_modules/recharts/es6/component/TooltipBoundingBox.js (12:113)
_inherits
node_modules/recharts/es6/component/TooltipBoundingBox.js (53:3)
PureComponent
node_modules/recharts/es6/component/TooltipBoundingBox.js (156:3)
(rsc)/./node_modules/recharts/es6/component/TooltipBoundingBox.js
file:///Users/yav1n/mediakit/.next/server/vendor-chunks/recharts.js (280:1)
Next.js
eval
webpack-internal:/(rsc)/node_modules/recharts/es6/component/Tooltip.js
(rsc)/./node_modules/recharts/es6/component/Tooltip.js
file:///Users/yav1n/mediakit/.next/server/vendor-chunks/recharts.js (270:1)
Next.js
eval
webpack-internal:/(rsc)/node_modules/recharts/es6/chart/generateCategoricalChart.js
(rsc)/./node_modules/recharts/es6/chart/generateCategoricalChart.js
file:///Users/yav1n/mediakit/.next/server/vendor-chunks/recharts.js (170:1)
Next.js
eval
webpack-internal:/(rsc)/node_modules/recharts/es6/chart/LineChart.js
(rsc)/./node_modules/recharts/es6/chart/LineChart.js
file:///Users/yav1n/mediakit/.next/server/vendor-chunks/recharts.js (160:1)
Next.js
eval
webpack-internal:/(rsc)/src/components/MediaKitPage.tsx
(rsc)/./src/components/MediaKitPage.tsx
file:///Users/yav1n/mediakit/.next/server/app/page.js (162:1)
Next.js
eval
webpack-internal:/(rsc)/src/app/page.tsx
(rsc)/./src/app/page.tsx
file:///Users/yav1n/mediakit/.next/server/app/page.js (151:1)
Next.js
The issue is related to server-side rendering in Next.js. Let's modify our component to use dynamic imports for Recharts and render it only on the client side. Here's how we'll fix it:
- First, we created a new file
src/components/TwitchStats.tsx
- Separated the Recharts component into its own file
- Added 'use client' directive to the Recharts component
- Used dynamic import for the chart component
- Added a loading state for better UX
- Disabled SSR for the chart component
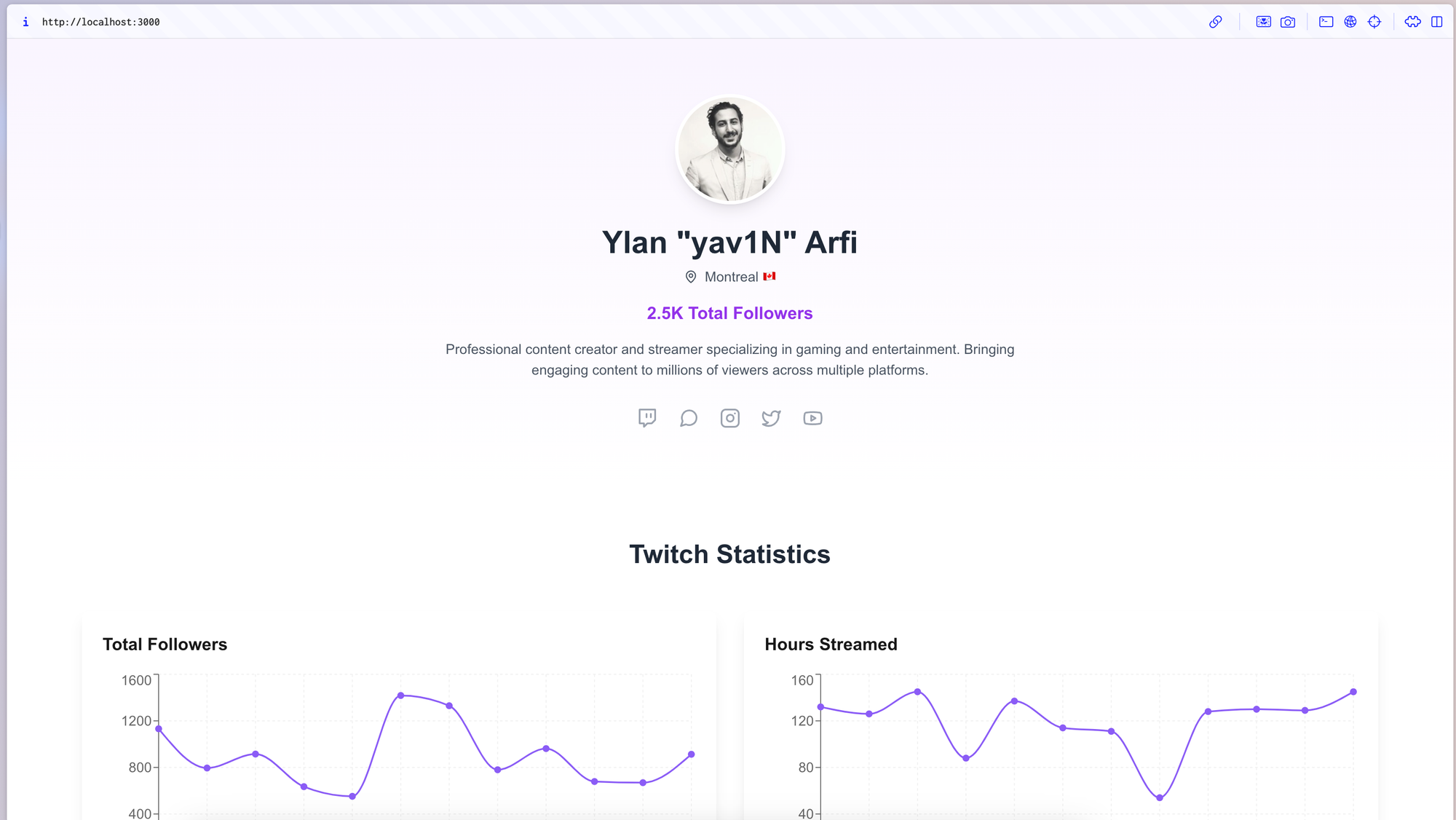