This file specifies intentionally untracked files or directories that Git should ignore when looking for changes to version. Entries in this file can be specified using patterns to match filenames, directories, or file paths.
Why is .gitignore
important for any Python project (or any project in general)?
- Unnecessary Files: When working with Python, files like
__pycache__
,.pyc
and others are generated. These files are either compilation caches or other runtime generated files which don't need to be in version control as they are derived from your source code and can be regenerated. - Environment Specific Configurations: If you have configuration files or environment variables stored in files that are specific to your development setup (like database configuration), you don't want these to be part of the version control system as they may differ across environments or could potentially leak sensitive information.
- Virtual Environments: It's common in Python development to use virtual environments to manage dependencies. The directories containing these virtual environments (
venv
or.env
for instance) can be quite large and shouldn't be added to the repository as they contain binaries and packages specific to one's setup. - Log files and Databases: Local log files, SQLite databases, or other generated data files are also candidates to be ignored. They can change frequently and are typically specific to a developer's individual environment or are just transient data.
- Avoid Clutter: By ignoring unnecessary files, the repository remains clean, making it easier to understand the project structure and preventing unwanted files from being committed by mistake.
- Reduce Repository Size: By excluding binaries and unnecessary files, the size of the repository is kept to a minimum. This makes cloning and fetching from the repo faster.
- Conflicts Avoidance: Frequently changed but unimportant files (like logs) can cause merge conflicts if they are checked into version control. Ignoring them prevents such conflicts.
A Sample .gitignore
for Python Projects:
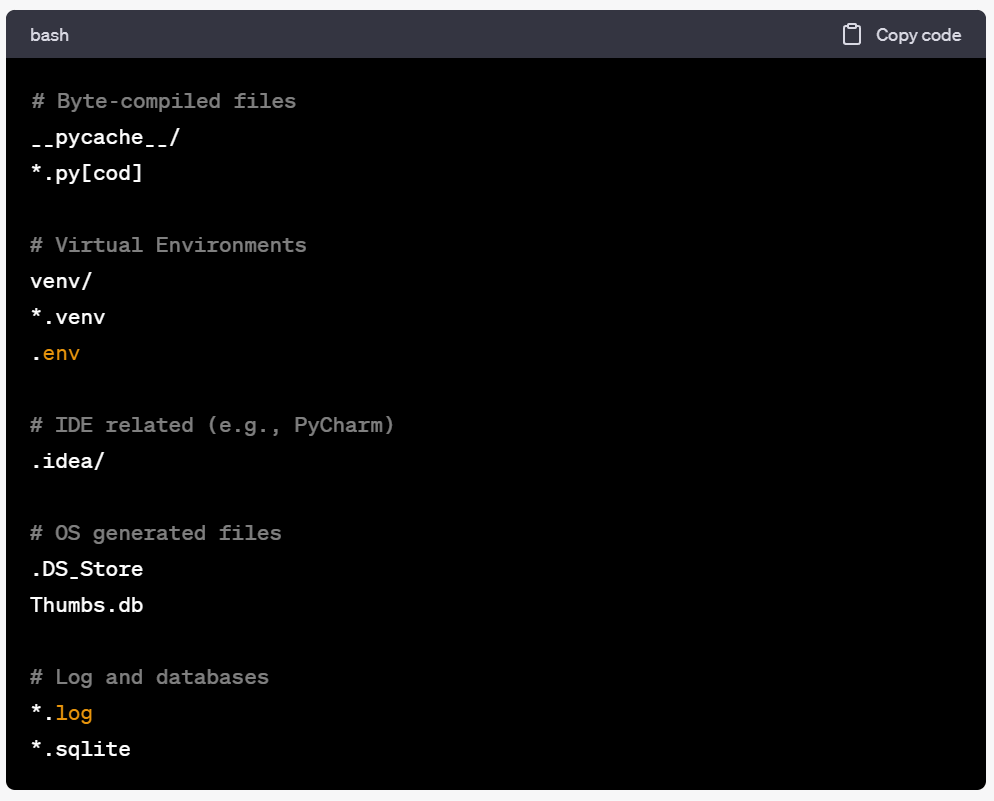
By making sure you have a well-configured .gitignore
file in your Python projects (or any project), you ensure that only relevant, necessary files are committed, making your repository cleaner, more secure, and more manageable.
.gitignore
can vary based on what tools, frameworks, and environments you're using. However, I'll provide a comprehensive .gitignore
file that caters to several common scenarios, especially for Python developers. Depending on your project, you might want to tailor this to your needs.
Let's walk through this .gitignore
file.
Byte-compiled files
__pycache__/
*.py[cod]
*$py.class
Python compiles its source code into byte code, which is then executed by the Python runtime. These compiled files are stored in the __pycache__
directory and have extensions like .pyc
, .pyo
, or .pyd
. $py.class
files are created when running Jython (Python on the JVM). We ignore them because they are generated and don't need to be version-controlled.
C extensions
*.so
Shared object files (*.so
) are binary files produced when building Python C extensions. They are platform-specific and should not be version-controlled.
Distribution / packaging
.Python
build/
develop-eggs/
dist/
downloads/
eggs/
.eggs/
lib/
lib64/
parts/
sdist/
var/
wheels/
share/python-wheels/
*.egg-info/
.installed.cfg
*.egg
These folders and files relate to Python's packaging ecosystem. When you build or install packages, these directories may get created. They store temporary build artifacts, package distributions, eggs (a previous Python distribution format), and more. They are generated and not needed in version control.
PyInstaller
*.manifest
*.spec
PyInstaller is a tool for creating standalone executables from Python applications. These files are specific to the packaging process and aren't necessary for version control.
Installer logs
pip-log.txt
pip-delete-this-directory.txt
pip
is Python's package installer. These files are logs and temporary directories used by older versions of pip
.
Unit test / coverage reports
htmlcov/
.tox/
.nox/
.coverage
.coverage.*
.cache
nosetests.xml
coverage.xml
*.cover
*.py,cover
.hypothesis/
.pytest_cache/
These are directories and files created by testing and coverage tools, like pytest
, nose
, tox
, nox
, and others. They store test results, virtual environments for testing, and cached data.
Translations
*.mo
*.pot
These files relate to internationalization (i18n) and localization (l10n) of Python applications. They store compiled translation files and templates.
Django, Flask, Scrapy, and other frameworks
*.log
local_settings.py
db.sqlite3
db.sqlite3-journal
# Flask stuff:
instance/
.webassets-cache
# Scrapy stuff:
.scrapy
Various Python frameworks and libraries generate specific files and directories. For example, Django might produce log files, SQLite databases, and local settings, while Flask might create an instance
directory and web asset caches. Scrapy, a web scraping library, has its own cache directory.
Documentation and builders
docs/_build/
# PyBuilder
target/
When generating documentation with Sphinx, build artifacts are stored in docs/_build/
. PyBuilder, a build automation tool, creates a target/
directory.
Jupyter, IPython, and other development tools
.ipynb_checkpoints
# IPython
profile_default/
ipython_config.py
# pyenv
.python-version
# pipenv
#Pipfile.lock
Jupyter notebooks create checkpoint files for recovery. IPython, an interactive Python shell, has profiles and configuration files. pyenv
is a Python version manager and creates a .python-version
file. pipenv
manages both packages and Python versions, and while Pipfile.lock
usually should be committed, there are cases where it shouldn't be, so it's commented out here.
Various tools and libraries
# Celery stuff
celerybeat-schedule
celerybeat.pid
# SageMath parsed files
*.sage.py
# Environments
.venv/
env/
venv/
ENV/
env.bak/
venv.bak/
pythonenv*
# Spyder project settings
.spyderproject
.spyproject
# Rope project settings
.ropeproject
# mkdocs documentation
/site
# mypy
.mypy_cache/
.dmypy.json dmypy.json
# Pyre type checker
.pyre/
# pytype static type analyzer
.pytype/
# Cython debug symbols
cython_debug/
This section covers a range of tools and libraries. For example:
pyflow
uses__pypackages__
directories.- Celery, an asynchronous task queue, creates schedules and PID files.
- Virtual environments store dependencies and can be created using
virtualenv
orvenv
. - Spyder, an IDE, and Rope, a refactoring library, have their own project files.
- Static type checkers and analyzers like
mypy
,pyre
, andpytype
generate cache and config files. - Cython, which compiles Python to C, produces debug symbols.
OS-specific files and global ignores
.vscode/
# Jetbrains IDEs (PyCharm, IntelliJ)
.idea/
# macOS
.DS_Store
# Windows image file caches
Thumbs.db
ehthumbs.db
# Folder config file
Desktop.ini
# Recycle Bin used on file shares
$RECYCLE.BIN/
# Linux *
.swp *
.swo
# Large media files (videos, audio, large datasets)
*.mp4
*.mp3
*.flac
*.wav
*.zip
*.tar.gz
*.data
# dotenv environment variable files
.env
.env.test
# Temporary files
*.tmp
*.bak
*.swp
*.old
*.save
# Log files
*.log
This section is for files and directories that aren't Python-specific:
- Editor/IDE configurations (
vscode
, Jetbrains' IDEs). - OS-specific files like macOS's
.DS_Store
, Windows thumbnails, and Linux swap files. - General files we might want to ignore, like large media files, environment variable configurations, temporary files, and log files.
This is an extensive .gitignore
file, and for most projects, not all of these lines will be necessary. Still, it provides a comprehensive starting point. Tailor it to your specific project and needs!